Reps 'N Recipes
A comprehensive fitness application that combines workout tracking and nutrition management, helping users achieve their health and wellness goals all in one place.
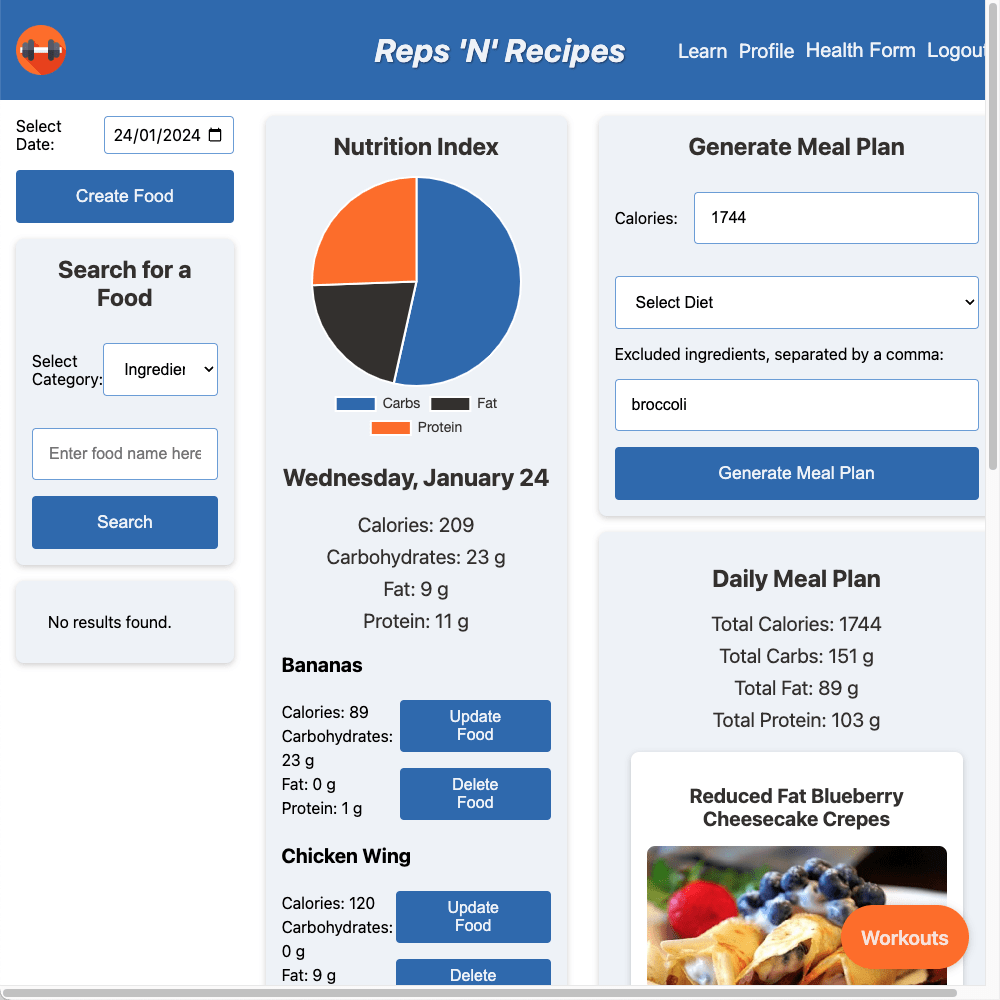
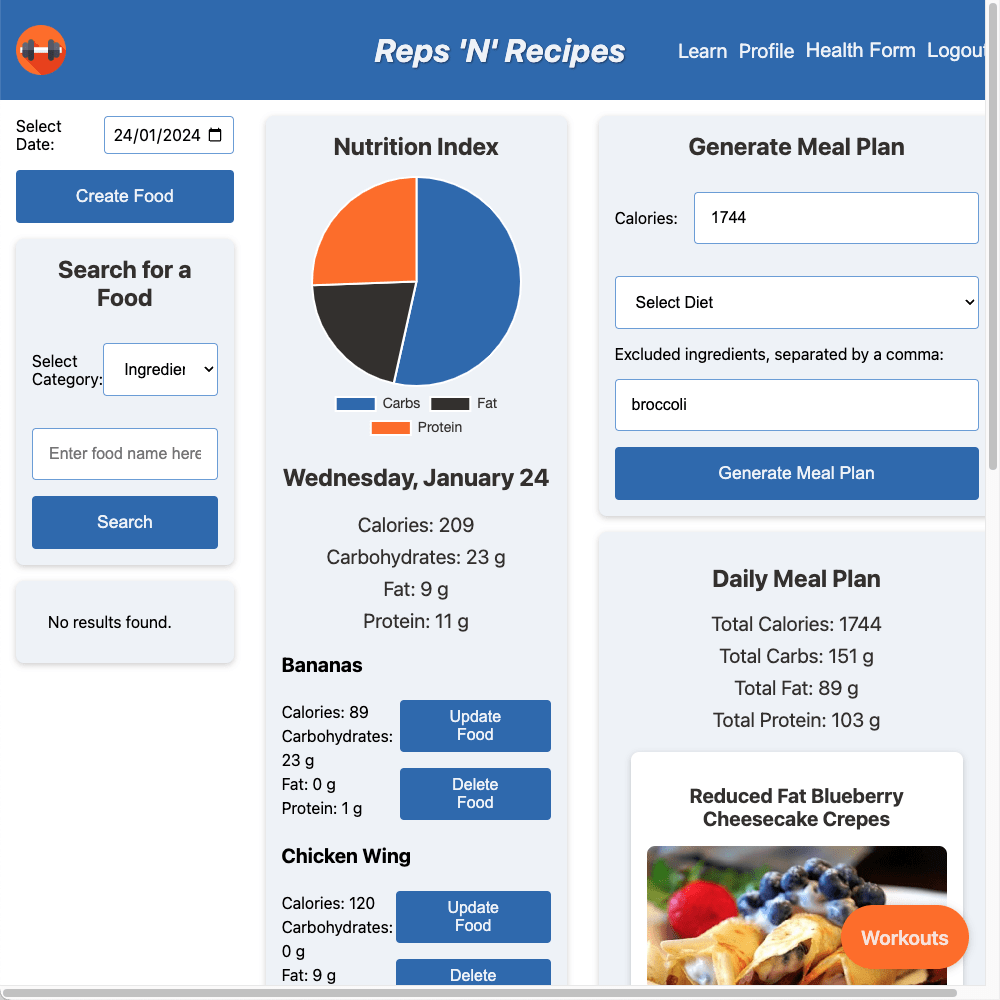
Project Overview
Reps 'N Recipes is a fitness app designed to help users achieve their health and wellness goals. While many fitness apps focus on either workout tracking or nutrition management, Reps 'N Recipes combines both features into one seamless application, eliminating the need for multiple apps.
The app features a database of built-in exercises with GIFs for proper form guidance, allows manual exercise input, and generates workouts for beginners. Additionally, it leverages the Spoonacular API for comprehensive meal tracking and can generate personalized meal plans based on user parameters.
Technology Stack
MERN Stack
Built with MongoDB, Express.js, React.js, and Node.js, creating a robust and scalable application architecture with a flexible NoSQL database.
State Management
Redux for centralized state management, particularly valuable for handling complex application state across workout and nutrition tracking features.
Data Visualization
Chart.js integrated with React to create interactive visualizations of user nutrition data, caloric intake, macros, weight tracking, and exercise progression over time.
External APIs
Spoonacular API for comprehensive food and nutrition data, including ingredients, products, recipes, menu items, and meal plan generation capabilities.
Cloud Storage
Amazon Web Services (AWS) S3 for scalable and secure storage of exercise demonstration GIFs, enhancing the user experience with visual guidance.
Key Features
User Profile & Customization
- • Personalized onboarding survey for physical measurements
- • Automated TDEE calculation and caloric recommendations
- • Goal setting and progress tracking
- • Weight updates with automatic TDEE adjustments
Workout Tracking
- • Pre-defined workout programs for beginners
- • Custom workout creation with exercise library
- • Exercise GIFs for proper form guidance
- • Set and rep tracking with weight progression
Nutrition Tracking
- • Comprehensive food database via Spoonacular
- • Meal logging with portion adjustments
- • Daily macronutrient and calorie tracking
- • Calendar view for nutrition history
Data Analysis
- • Visual progress charts for key metrics
- • Weight tracking over time
- • Estimated 1-rep max calculations
- • Nutrition trend analysis
Feature Demos
Health Profile Setup
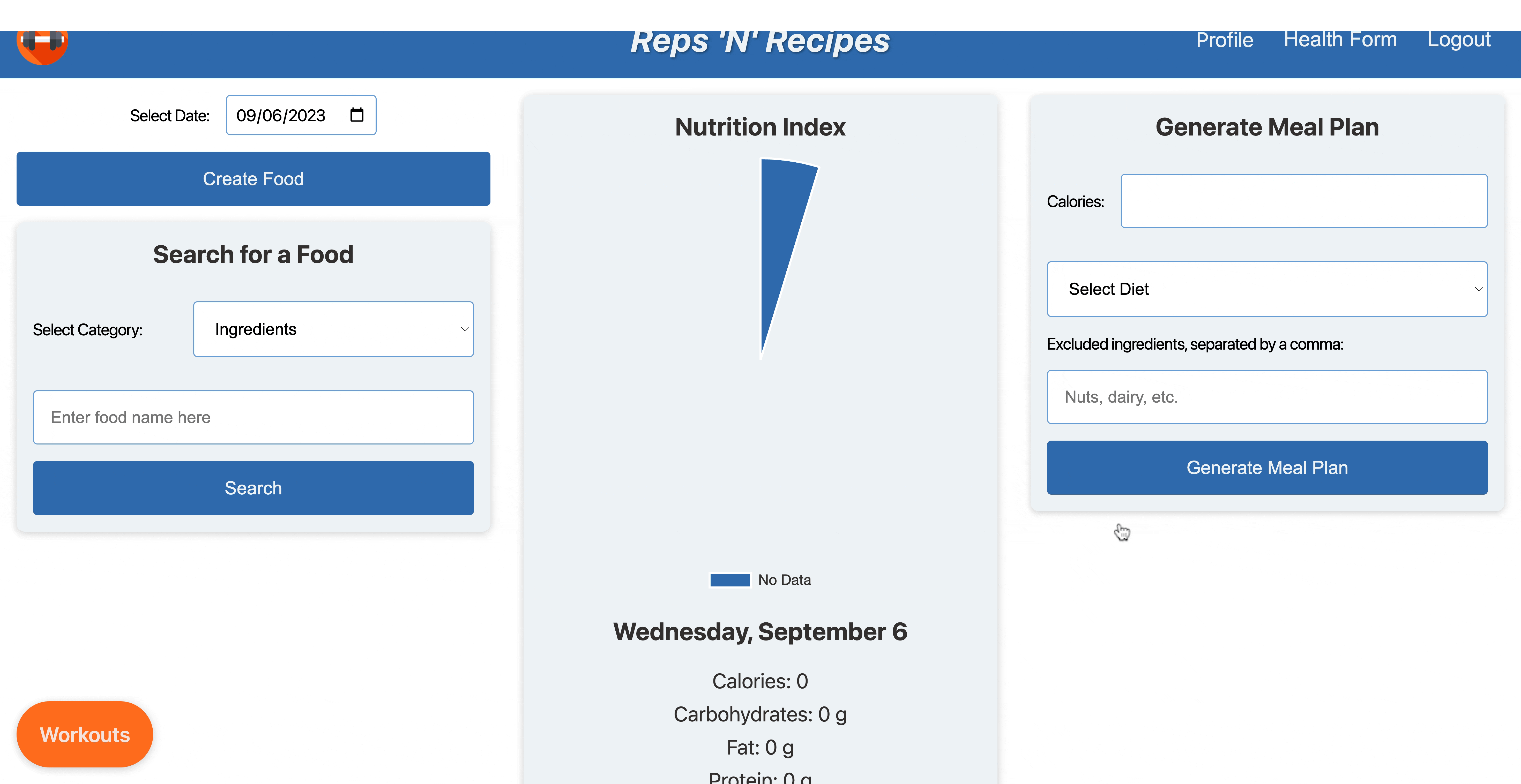
Workout Management
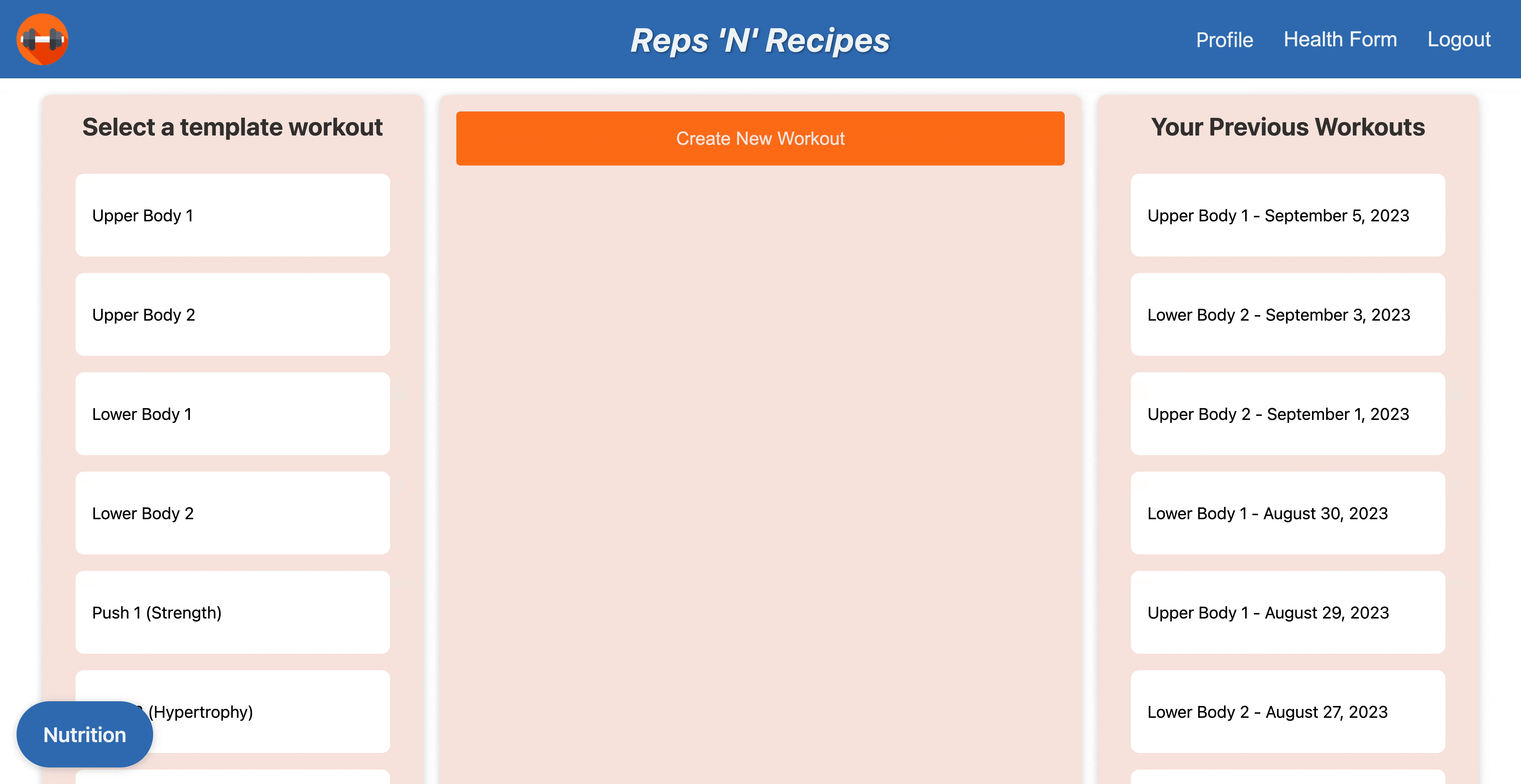
Nutrition Tracking
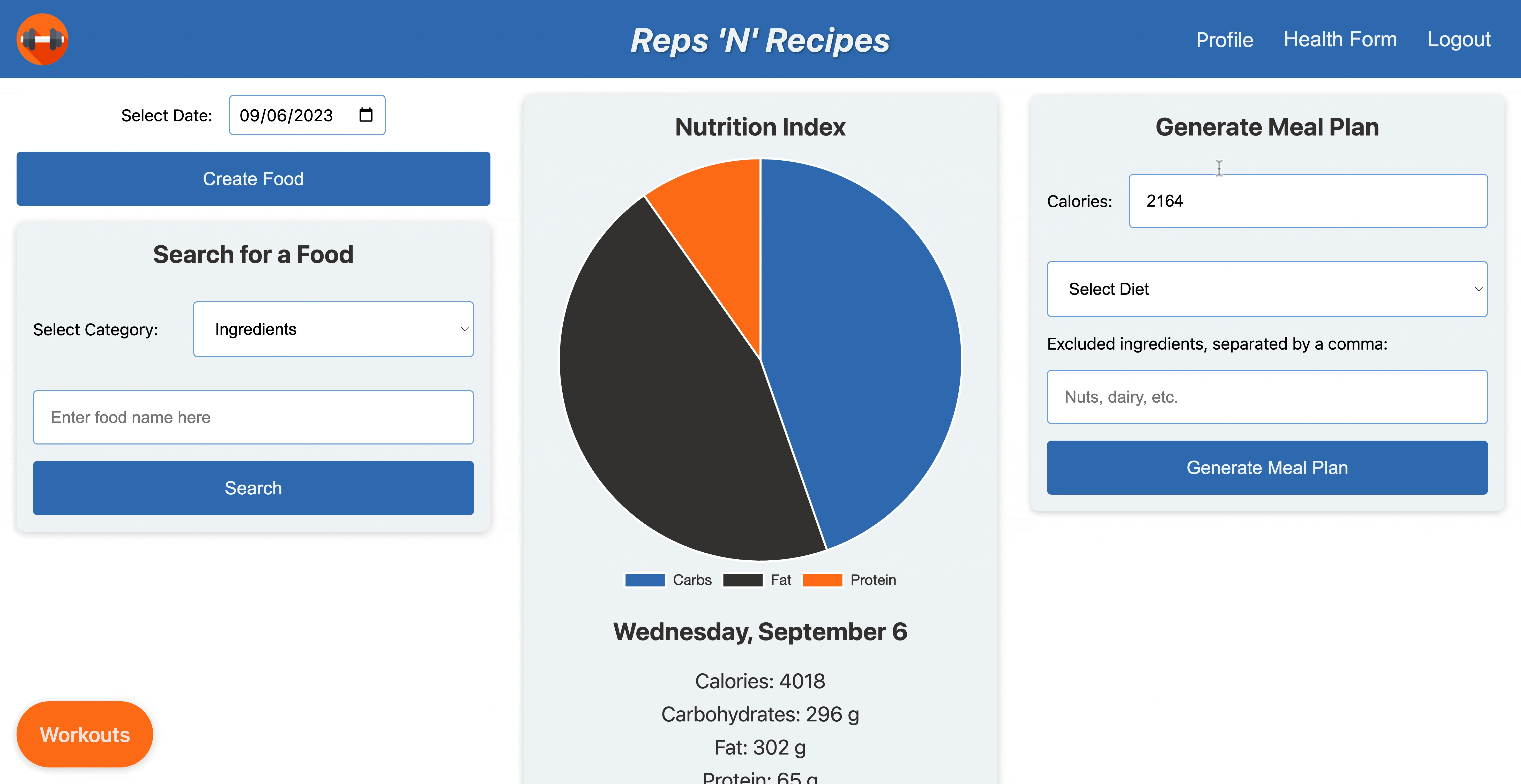
Progress Analytics
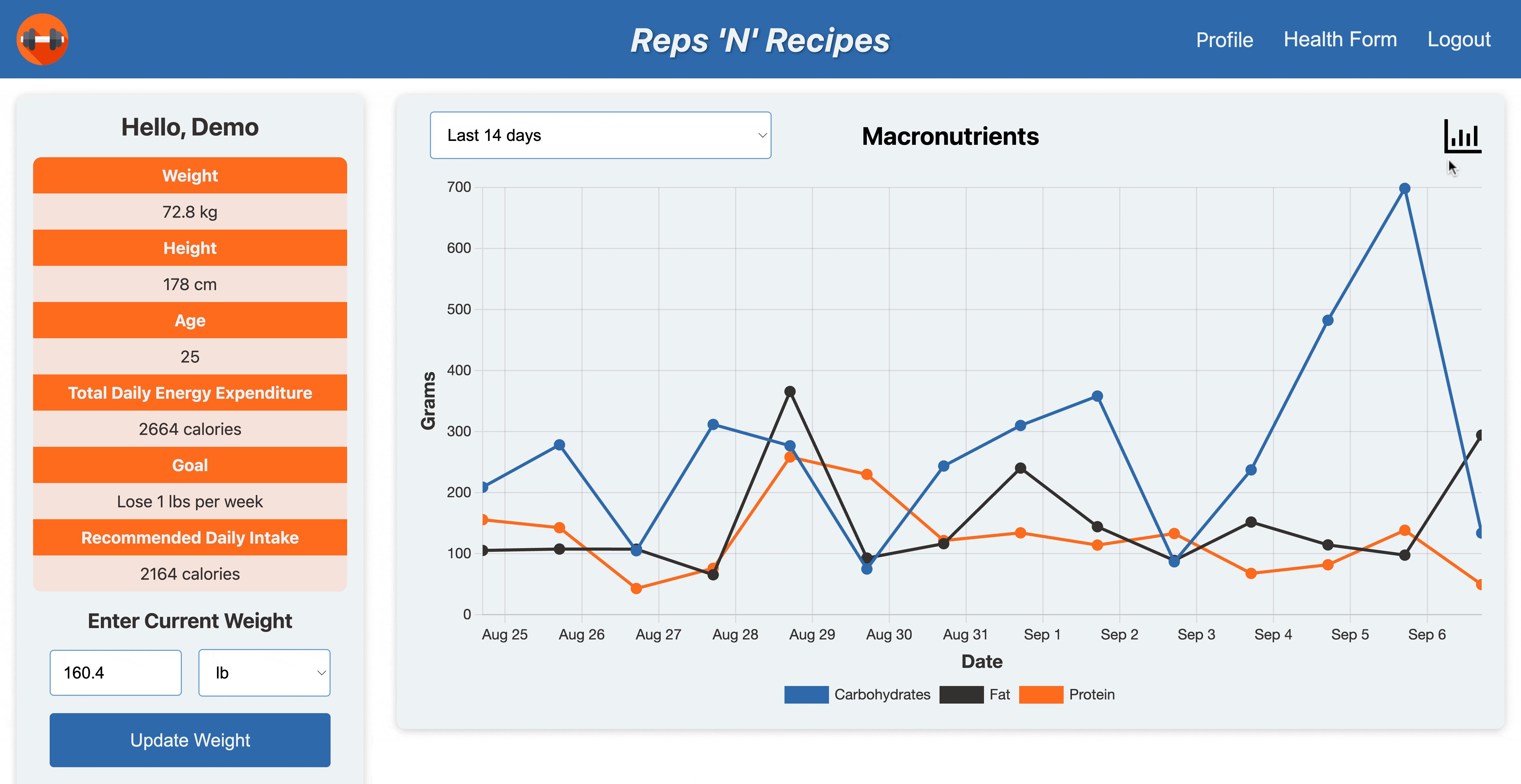