Fairbnb
A comprehensive full-stack Airbnb clone built with React/Redux frontend and Ruby on Rails backend, featuring Google Maps integration and AWS S3 for media storage.
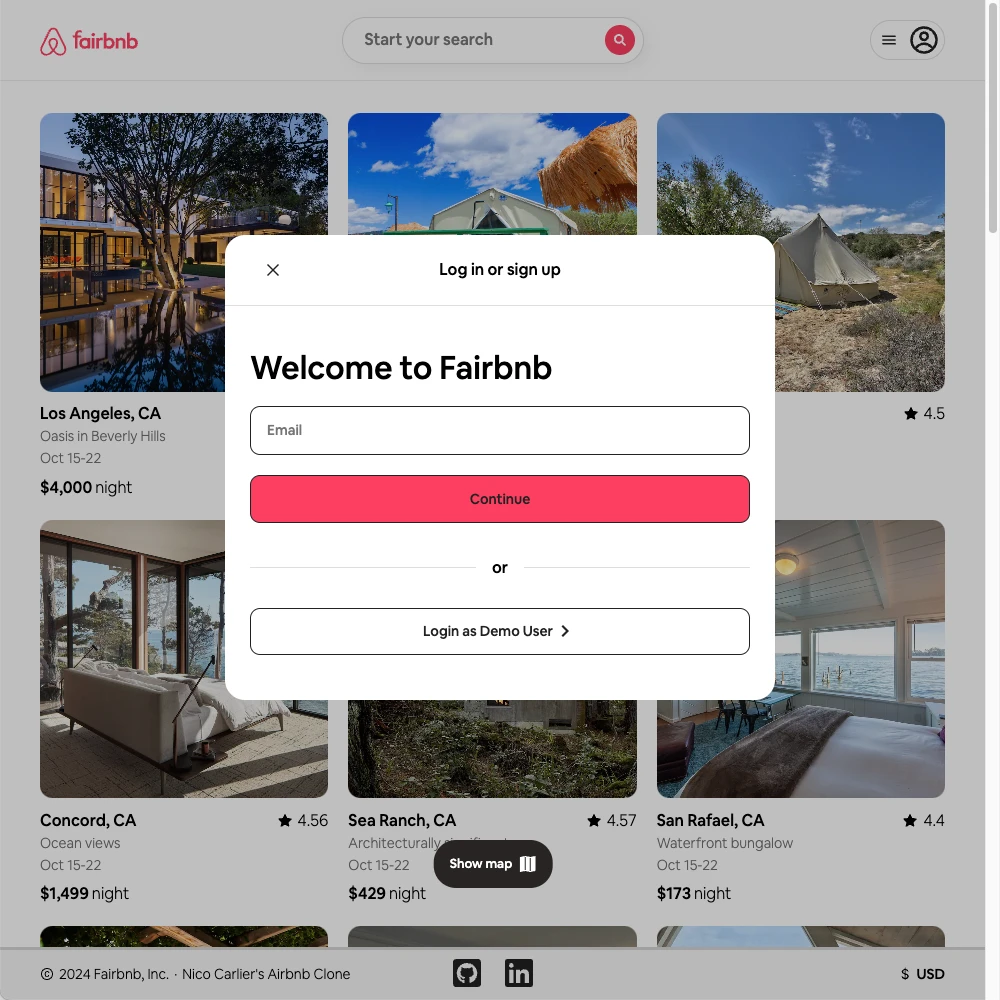
Project Overview
Fairbnb is a full-stack web application that meticulously emulates the Airbnb experience, allowing users to discover, explore, and book accommodations with ease. The platform combines a responsive React/Redux frontend with a Ruby on Rails backend to deliver a seamless and intuitive user experience.
I prioritized data integrity and user experience by implementing robust encapsulation principles, ensuring that users can only review properties they've stayed at, preventing post-experience booking modifications, and enforcing homeowner-defined constraints for all reservations.
Technology Stack & Rationale
Backend
Built with Ruby on Rails for its convention-over-configuration paradigm, accelerating development and providing excellent scalability for growing user bases. Rails' robust structure is particularly well-suited for platforms like Fairbnb that anticipate expanding data needs.
Database
PostgreSQL database with Active Record ORM, ensuring data integrity and enabling complex operations through object-oriented database interactions. This pairing excels at maintaining critical relational data like user profiles, property listings, and booking records.
Frontend
React.js with Redux for state management, creating a dynamic and modular UI with predictable state updates and reusable components. This architecture promotes code reusability and efficient rendering, essential for the interactive features that define the Fairbnb experience.
APIs & Libraries
Integrated Google Maps API for interactive location visualization, Date Range Picker for intuitive booking calendar functionality, and AWS S3 for optimized media storage and delivery, enhancing performance without compromising on functionality.
Key Features
User Authentication
- •Secure account management with CSRF protection and encrypted passwords
- •Intelligent email routing for streamlined signup/login flow
- •Comprehensive password strength validation with real-time feedback
- •Demo user access for quick platform exploration
Property Discovery
- •Interactive map integration with Google Maps API
- •Keyword search functionality for targeting specific amenities or locations
- •Immersive photo slideshows for comprehensive property previews
- •Expandable property descriptions and user reviews
Booking System
- •Interactive booking calendar with Date Range Picker integration
- •Real-time availability updates and conflict prevention
- •Dynamic pricing calculations based on duration and property rates
- •Comprehensive booking management interface for users
Review System
- •Post-stay review creation with data integrity validation
- •Encapsulated review system allowing only verified guests to leave reviews
- •Rating system with detailed feedback categories
- •Property listing review display with expandable details
Technical Challenges & Solutions
Date Handling Across Time Zones
When handling booking dates between the frontend and backend, timezone inconsistencies created significant challenges. Dates saved in the database as yyyy-mm-dd
strings would lose timezone data, causing potential date shifts when displayed in the user's browser.
Solution: UTC Standardization
I implemented a bidirectional conversion system that standardizes all dates to UTC before storage and converts them back to the user's local timezone upon retrieval:
export const convertLocalDateToUTC = (inputDate) => { const parts = inputDate.split("/"); const day = parseInt(parts[1], 10); const month = parseInt(parts[0], 10); const year = parseInt(parts[2], 10); const timeZone = Intl.DateTimeFormat().resolvedOptions().timeZone; const localDate = DateTime.fromObject({ day, month, year }).setZone(timeZone); const utcDate = localDate.toUTC(); return utcDate.toFormat('dd/MM/yyyy'); } export const UTCDateBooking = (booking) => { return {...booking, startDate: convertLocalDateToUTC(booking.startDate), endDate: convertLocalDateToUTC(booking.endDate) } }
This approach ensures consistent date handling regardless of user location or timezone settings, maintaining data integrity throughout the booking lifecycle.
Development Insights
Building Fairbnb provided valuable experience in full-stack development with a focus on data integrity and user experience. Key learnings included:
- Implementing proper encapsulation principles to maintain data integrity is crucial for booking platforms
- Managing date and time data requires thorough consideration of timezone implications
- Integrating third-party APIs like Google Maps and AWS S3 significantly enhances functionality while requiring thoughtful implementation
- Custom styling third-party components (like Date Range Picker) often requires creative approaches to override default behaviors
- A well-designed Redux store architecture is essential for managing complex application state in a predictable manner