NextChess
A full-stack chess platform built with Next.js and TypeScript, featuring real-time multiplayer gameplay, custom move validation engine, and responsive design for all devices.
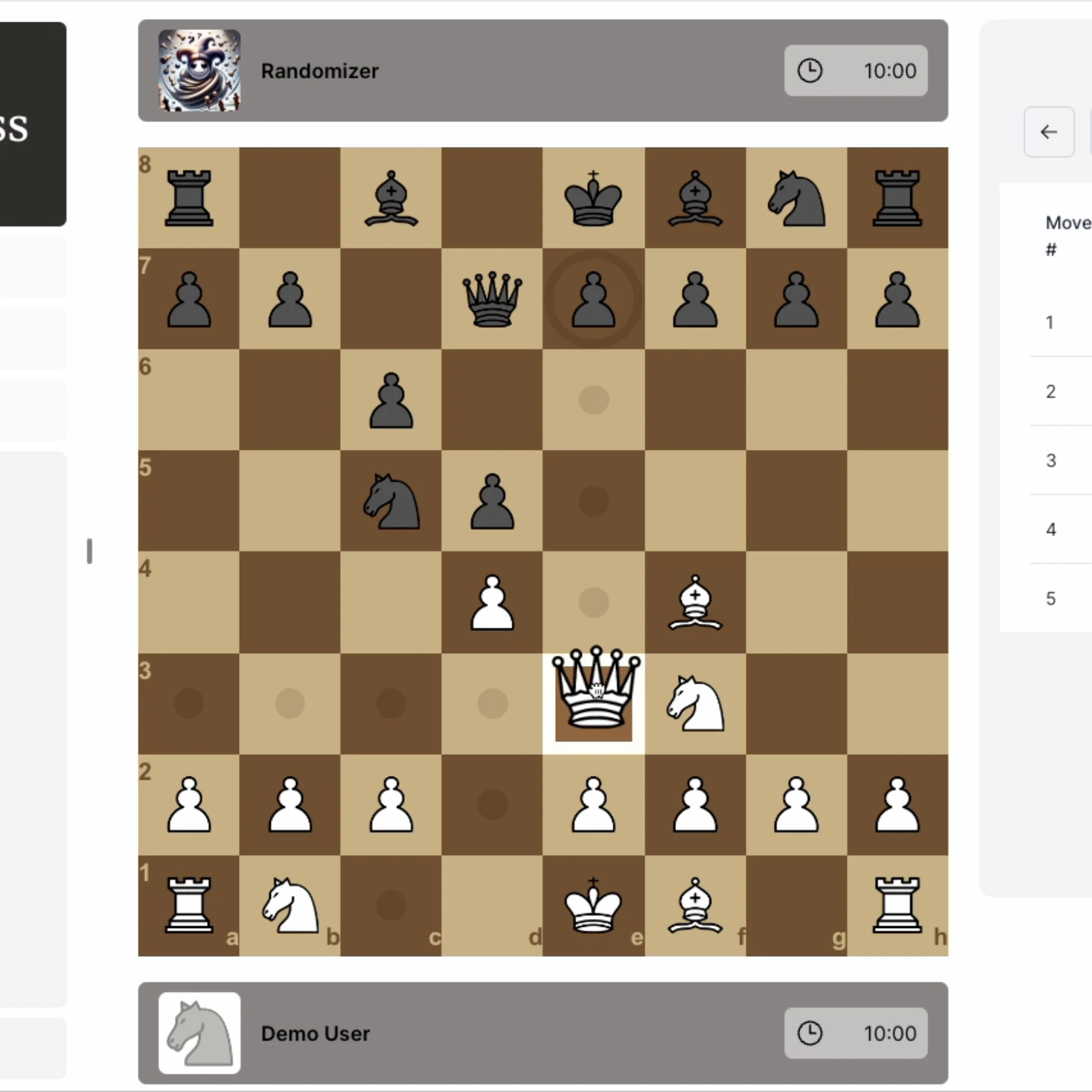
Project Overview
NextChess is a comprehensive chess platform that brings the classic game into the modern web era. Built with a focus on performance and user experience, it combines a custom chess engine with an intuitive interface to deliver an engaging gameplay experience for players of all skill levels.
The application offers real-time multiplayer matches, AI opponents, game history tracking, and responsive design that adapts seamlessly to any device. By leveraging Next.js 14 and TypeScript, the platform ensures type safety and optimal performance while maintaining clean, maintainable code.
Technology Stack & Rationale
Frontend & Backend
Built with Next.js 14, leveraging its full-stack capabilities for server-side rendering, API routes, and seamless page transitions. TypeScript provides robust type safety throughout the codebase, reducing runtime errors and improving developer experience.
State Management
Redux manages application state, providing a predictable state container for complex chess logic, game history, and user interactions. This architecture ensures consistent state across components and enables features like game replay and move validation.
Styling & Responsiveness
Tailwind CSS combined with CSS modules creates a responsive and visually appealing interface. The design system adapts gracefully from mobile to desktop, maintaining usability and aesthetic consistency across all screen sizes.
Real-time Communication
WebSocket integration enables real-time multiplayer gameplay with minimal latency. Players experience smooth, responsive interactions during matches, enhancing the competitive nature of the game.
Deployment
Vercel platform provides seamless deployment with automatic preview environments for each pull request, ensuring high availability and performance for users worldwide.
Key Features
Custom Chess Engine
- •Hand-crafted move validation system with specialized piece classes
- •FEN notation support for game state serialization
- •Advanced chess rules including en passant, castling, and promotion
- •Comprehensive check and checkmate detection
Multiplayer & AI
- •Real-time multiplayer matches with WebSocket communication
- •AI opponents powered by Stockfish integration
- •Multiple difficulty levels for AI gameplay
- •Matchmaking system for finding online opponents
Interactive UI
- •Custom drag-and-drop system optimized for chess piece movement
- •Visual move highlighting showing legal moves and captures
- •Interactive move history with algebraic notation
- •Game state indicators for check, checkmate, and draw conditions
User Experience
- •Secure authentication via GitHub and Google OAuth
- •Responsive design optimized for mobile, tablet, and desktop
- •Game history and statistics tracking
- •Light and dark mode themes
Technical Challenges & Solutions
Performance Optimization
One of the most significant challenges was managing component re-renders in the chessboard. With 64 squares and multiple pieces, even small state changes would trigger cascading re-renders, impacting performance and user experience.
Problem: DragClone Excessive Re-rendering
The DragClone
component's position state changes were causing unnecessary re-renders of all child components, even when using React's memoization techniques.
const usePreviousProps = (props) => { const ref = useRef(); useEffect(() => { ref.current = props; }); return ref.current; // Returns the previous props };
I implemented a custom hook to compare prop changes between renders, which helped identify playMoveIfValid
as the volatile prop. Further analysis revealed unstable dependencies in useCallback hooks for game state management functions.
Solution: Strategic Memoization
The solution involved multiple optimization techniques:
- Ensuring dependency stability in useCallback and useMemo hooks
- Implementing React.memo for chess square components with custom comparison functions
- Separating drag state management from board rendering logic
- Using useRef for position tracking to avoid unnecessary state updates
Chess Logic Implementation
Building a robust chess engine required careful consideration of the game's complex rules, particularly for special moves like castling and detecting check/checkmate conditions.
Object-Oriented Design with Inheritance
I implemented a class-based structure for chess pieces, using inheritance and composition to share common behaviors while allowing piece-specific move logic:
import { inherit } from './inherit.js'; import { Piece } from './piece.js'; import { Slideable } from './slideable.js'; const DIRS = [[1,0],[0,1],[-1,0],[0,-1],[1,1],[-1,-1],[-1,1],[1,-1]]; export function Queen(color, square, board) { this.pieceName = "queen"; this.type = color.slice(0,1) + "_" + this.pieceName; Piece.call(this, color, square, board); this.slideable = new Slideable(board); this.fenChar = color === "white" ? "Q" : "q"; } inherit(Piece, Queen); Queen.prototype.pieceMoves = function(pos = this.getPos()) { const color = this.getColor(); const [options, takeOptions] = this.slideable.calculateMoves(color, pos, DIRS); return [options, takeOptions]; }
This approach allowed for efficient code reuse while maintaining the unique movement patterns of each chess piece. The Slideable composition pattern was particularly effective for handling the line-based movements of queens, rooks, and bishops.
Development Insights
Building NextChess provided valuable experience in both frontend and game development. Key learnings included:
- Performance optimization is critical for interactive applications, especially those with complex state management
- Object-oriented design principles translate effectively to JavaScript/TypeScript when building complex systems
- FEN notation provides an elegant solution for chess game state serialization
- WebSockets enable truly real-time experiences that traditional HTTP requests cannot match
- Careful component architecture planning pays dividends when scaling application features
Future Enhancements
While NextChess is fully functional, several enhancements are planned for future iterations:
- Player rankings and ELO system for competitive play
- Tournament functionality with brackets and time controls
- Puzzle mode for chess training and skill improvement
- Opening book integration for learning standard chess openings
- Advanced analysis tools with position evaluation